- Home
- Integration Frameworks
- Apache Camel
- Getting started with Apache Camel using Java
Apache Camel is a very useful library that helps you process events or messages from many different sources
Here I will provide a tutorial on how you can get started with Apache Camel using Java. If you have idea on mule ESB, then easy to understand Apache camel..
Important elements in Apache Camel
Router
Routers play a crucial role in controlling the trajectory a message will follow when it transits. They're the gatekeepers of the endpoints of a service. In fact, they act like railroad switches, taking care of keeping messages on the right succession of tracks so they can reach their intended destinations.
Transformer
A transformer optionally changes incoming or outgoing messages in some way.This is usually done to make the message format useable by a downstream function.
Bean/Component
Components are usually business objects. They are components that execute business logic on an incoming event. Components are standard JavaBeans (containers).
Below class is Camel Router class, using this I am trying to achieve copying files one location to other location within time period. Whenever any file reaches to input location, will be copied to output location..
Using transformer content can be transform to other format, process related can be handled by processor and logic can be handled by bean(component)..
Router class
package com.javavillage.firstcamelprj;
import org.apache.camel.builder.RouteBuilder;
public class FirstRouteBuilder extends RouteBuilder{
@Override
public void configure() throws Exception {
from("file:F:/camel/input?noop=true")
.process(new LogProcessor())
.bean(new Transormer(),"transformContent")
.to("file:F:/camel/output");
}
}
Processor
package com.javavillage.firstcamelprj;
import org.apache.camel.Exchange;
import org.apache.camel.Processor;
public class LogProcessor implements Processor{
public void process(Exchange exchange) throws Exception {
System.out.println("processing "+exchange.getIn().getBody(String.class));
}
}
Transformer: Retrieving content and changing to uppercase
package com.javavillage.firstcamelprj;
public class Transormer {
public String transformContent(String body)
{
System.out.println("invoking the transformContent method");
String upperCaseContent=body.toUpperCase();
//System.out.println(object);
return upperCaseContent;
}
}
Maven entries for pom.xml
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.5</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.5</version>
</dependency>
Below is my application execution
package com.javavillage.firstcamelprj;
import org.apache.camel.CamelContext;
import org.apache.camel.impl.DefaultCamelContext;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args )
{
FirstRouteBuilder routeBuilder=new FirstRouteBuilder();
CamelContext ctx=new DefaultCamelContext();
try {
ctx.addRoutes(routeBuilder);
ctx.start();
Thread.sleep(5*60*1000);
ctx.stop();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Apache Camel Application Structure

Execute Apache Camel Application:
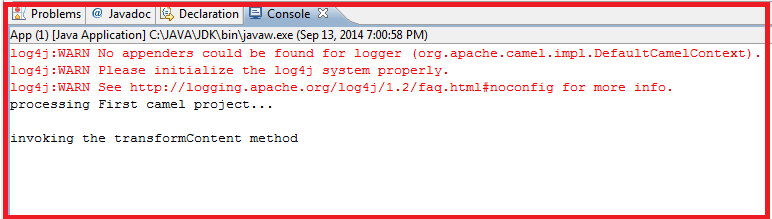
Paste a file at input location and check console as well output location.We can observe file transfor input folder to output folder